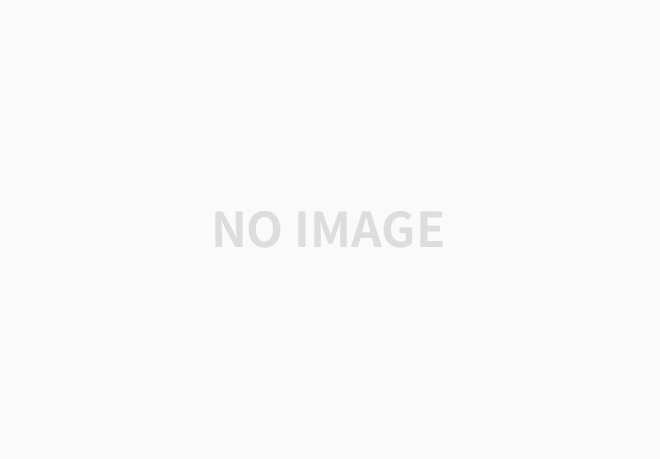
1. Vue 프로젝트 생성
Vue CLI를 사용하여 새로운 프로젝트를 생성합니다.
# Vue CLI가 설치되어 있지 않다면 먼저 설치합니다.
npm install -g @vue/cli
# 새로운 Vue 프로젝트를 생성합니다.
vue create my-vue-app
프로젝트 생성 시, 기본 설정을 선택하거나 커스텀 설정을 사용할 수 있습니다. 여기서는 기본 설정을 선택한다고 가정하겠습니다.
cd my-vue-app
npm run serve
npm run serve 명령을 실행하면, 로컬 개발 서버가 시작되고 기본적으로 http://localhost:8080에서 애플리케이션을 확인할 수 있습니다.
반응형
2. 프로젝트 구조
프로젝트 폴더 구조는 다음과 같습니다.
my-vue-app/
├── node_modules/
├── public/
│ └── index.html
├── src/
│ ├── assets/
│ ├── components/
│ ├── views/
│ ├── App.vue
│ ├── main.js
├── .gitignore
├── babel.config.js
├── package.json
└── README.md
3. 주요 파일 및 디렉토리 설명
- public/index.html: 애플리케이션의 기본 HTML 파일입니다.
- src/main.js: 애플리케이션의 진입점 파일로, Vue 인스턴스를 생성합니다.
- src/App.vue: 최상위 컴포넌트입니다.
- src/components/: 재사용 가능한 Vue 컴포넌트를 저장하는 디렉토리입니다.
4. App.vue 파일
<template>
<div id="app">
<img alt="Vue logo" src="./assets/logo.png">
<HelloWorld msg="Welcome to Your Vue.js App"/>
</div>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
5. HelloWorld 컴포넌트
기본적으로 생성된 HelloWorld 컴포넌트는 src/components/HelloWorld.vue에 위치합니다.
<template>
<div class="hello">
<h1>{{ msg }}</h1>
<p>
For a guide and recipes on how to configure / customize this project,<br>
check out the
<a href="https://cli.vuejs.org" target="_blank" rel="noopener">vue-cli documentation</a>.
</p>
</div>
</template>
<script>
export default {
name: 'HelloWorld',
props: {
msg: String
}
}
</script>
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
6. 데이터 바인딩과 이벤트 처리
데이터 바인딩 예시
App.vue 파일에서 간단한 데이터 바인딩을 추가해봅니다.
<template>
<div id="app">
<input v-model="message" placeholder="메시지를 입력하세요">
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
name: 'App',
data() {
return {
message: ''
}
}
}
</script>
이벤트 처리 예시
버튼을 클릭할 때 메시지를 변경하는 예시입니다.
<template>
<div id="app">
<button v-on:click="changeMessage">메시지 변경</button>
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
name: 'App',
data() {
return {
message: 'Hello Vue!'
}
},
methods: {
changeMessage() {
this.message = '메시지가 변경되었습니다!';
}
}
}
</script>
7. 컴포넌트 통신
부모 컴포넌트에서 자식 컴포넌트로 데이터를 전달하는 예시입니다.
부모 컴포넌트 (App.vue)
<template>
<div id="app">
<ChildComponent :parentMessage="message" />
</div>
</template>
<script>
import ChildComponent from './components/ChildComponent.vue'
export default {
name: 'App',
components: {
ChildComponent
},
data() {
return {
message: 'Hello from parent!'
}
}
}
</script>
자식 컴포넌트 (ChildComponent.vue)
<template>
<div>
<p>{{ parentMessage }}</p>
</div>
</template>
<script>
export default {
name: 'ChildComponent',
props: {
parentMessage: String
}
}
</script>
이 예시들을 통해 Vue.js의 기본적인 문법과 기능을 이해할 수 있습니다. Vue CLI를 사용하면 프로젝트 설정이 간편해지고, 다양한 Vue 기능을 쉽게 사용할 수 있습니다. Vue.js 공식 문서와 다양한 예제를 참고하여 더 많은 기능을 학습할 수 있습니다.
참고 문서
https://v2.ko.vuejs.org/v2/guide
시작하기 — Vue.js
Vue.js - 프로그레시브 자바스크립트 프레임워크
v2.ko.vuejs.org
https://v3-docs.vuejs-korea.org/guide/introduction.html
소개 | Vue.js
v3-docs.vuejs-korea.org
728x90